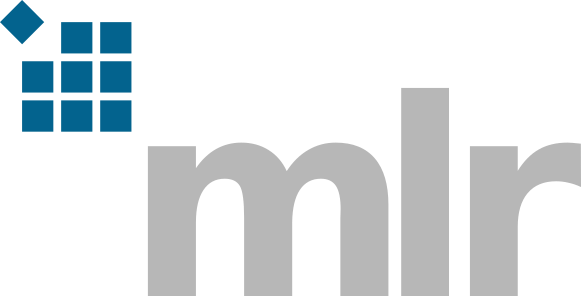
Hyperparameter Tuning with Design Points
Source:R/TunerBatchDesignPoints.R
mlr_tuners_design_points.Rd
Subclass for tuning w.r.t. fixed design points.
We simply search over a set of points fully specified by the user. The points in the design are evaluated in order as given.
Parallelization
In order to support general termination criteria and parallelization, we
evaluate points in a batch-fashion of size batch_size
. Larger batches mean
we can parallelize more, smaller batches imply a more fine-grained checking
of termination criteria. A batch contains of batch_size
times resampling$iters
jobs.
E.g., if you set a batch size of 10 points and do a 5-fold cross validation, you can
utilize up to 50 cores.
Parallelization is supported via package future (see mlr3::benchmark()
's
section on parallelization for more details).
Logging
All Tuners use a logger (as implemented in lgr) from package
bbotk.
Use lgr::get_logger("bbotk")
to access and control the logger.
Optimizer
This Tuner is based on bbotk::OptimizerBatchDesignPoints which can be applied on any black box optimization problem. See also the documentation of bbotk.
Parameters
batch_size
integer(1)
Maximum number of configurations to try in a batch.design
data.table::data.table
Design points to try in search, one per row.
Resources
There are several sections about hyperparameter optimization in the mlr3book.
Getting started with hyperparameter optimization.
An overview of all tuners can be found on our website.
Tune a support vector machine on the Sonar data set.
Learn about tuning spaces.
Estimate the model performance with nested resampling.
Learn about multi-objective optimization.
Simultaneously optimize hyperparameters and use early stopping with XGBoost.
Automate the tuning.
The gallery features a collection of case studies and demos about optimization.
Learn more advanced methods with the Practical Tuning Series.
Learn about hotstarting models.
Run the default hyperparameter configuration of learners as a baseline.
Use the Hyperband optimizer with different budget parameters.
The cheatsheet summarizes the most important functions of mlr3tuning.
Progress Bars
$optimize()
supports progress bars via the package progressr
combined with a Terminator. Simply wrap the function in
progressr::with_progress()
to enable them. We recommend to use package
progress as backend; enable with progressr::handlers("progress")
.
See also
Package mlr3hyperband for hyperband tuning.
Other Tuner:
Tuner
,
mlr_tuners
,
mlr_tuners_cmaes
,
mlr_tuners_gensa
,
mlr_tuners_grid_search
,
mlr_tuners_internal
,
mlr_tuners_irace
,
mlr_tuners_nloptr
,
mlr_tuners_random_search
Super classes
mlr3tuning::Tuner
-> mlr3tuning::TunerBatch
-> mlr3tuning::TunerBatchFromOptimizerBatch
-> TunerBatchDesignPoints
Examples
# Hyperparameter Optimization
# load learner and set search space
learner = lrn("classif.rpart",
cp = to_tune(1e-04, 1e-1),
minsplit = to_tune(2, 128),
minbucket = to_tune(1, 64)
)
# create design
design = mlr3misc::rowwise_table(
~cp, ~minsplit, ~minbucket,
0.1, 2, 64,
0.01, 64, 32,
0.001, 128, 1
)
# run hyperparameter tuning on the Palmer Penguins data set
instance = tune(
tuner = tnr("design_points", design = design),
task = tsk("penguins"),
learner = learner,
resampling = rsmp("holdout"),
measure = msr("classif.ce")
)
# best performing hyperparameter configuration
instance$result
#> cp minbucket minsplit learner_param_vals x_domain classif.ce
#> <num> <num> <num> <list> <list> <num>
#> 1: 0.01 32 64 <list[4]> <list[3]> 0.07826087
# all evaluated hyperparameter configuration
as.data.table(instance$archive)
#> cp minbucket minsplit classif.ce runtime_learners timestamp
#> <num> <num> <num> <num> <num> <POSc>
#> 1: 0.100 64 2 0.09565217 0.006 2024-12-18 10:06:55
#> 2: 0.010 32 64 0.07826087 0.007 2024-12-18 10:06:55
#> 3: 0.001 1 128 0.07826087 0.005 2024-12-18 10:06:55
#> warnings errors x_domain batch_nr resample_result
#> <int> <int> <list> <int> <list>
#> 1: 0 0 <list[3]> 1 <ResampleResult>
#> 2: 0 0 <list[3]> 2 <ResampleResult>
#> 3: 0 0 <list[3]> 3 <ResampleResult>
# fit final model on complete data set
learner$param_set$values = instance$result_learner_param_vals
learner$train(tsk("penguins"))